十四、对于boolean值,避免不必要的等式判断
将一个boolean值与一个true比较是一个恒等操作(直接返回该boolean变量的值). 移走对于boolean的不必要操作至少会带来2个好处
1)代码执行的更快 (生成的字节码少了5个字节);
2)代码也会更加干净 。
例子
public class UEQ
{
boolean method (String string) {
return string.endsWith ("a") == true; // Violation
}
}
更正
class UEQ_fixed
{
boolean method (String string) {
return string.endsWith ("a");
}
}
十五、对于常量字符串,用'String' 代替 'StringBuffer'
常量字符串并不需要动态改变长度。
例子
public class USC {
String method () {
StringBuffer s = new StringBuffer ("Hello");
String t = s + "World!";
return t;
}
}
更正
把StringBuffer换成String,如果确定这个String不会再变的话,这将会减少运行开销提高性能。
十六、用'StringTokenizer' 代替 'indexOf()' 和'substring()'
字符串的分析在很多应用中都是常见的。使用indexOf()和substring()来分析字符串容易导致StringIndexOutOfBoundsException。而使用StringTokenizer类来分析字符串则会容易一些,效率也会高一些。
例子
public class UST {
void parseString(String string) {
int index = 0;
while ((index = string.indexOf(".", index)) != -1) {
System.out.println (string.substring(index, string.length()));
}
}
}
参考资料
Graig Larman, Rhett Guthrie: "Java 2 Performance and Idiom Guide"
Prentice Hall PTR, ISBN: 0-13-014260-3 pp. 282 – 283
十七、使用条件操作符替代"if (cond) return; else return;" 结构
条件操作符更加的简捷
例子
public class IF {
public int method(boolean isDone) {
if (isDone) {
return 0;
} else {
return 10;
}
}
}
更正
public class IF {
public int method(boolean isDone) {
return (isDone ? 0 : 10);
}
}
十八、使用条件操作符代替"if (cond) a = b; else a = c;" 结构
例子
public class IFAS {
void method(boolean isTrue) {
if (isTrue) {
_value = 0;
} else {
_value = 1;
}
}
private int _value = 0;
}
更正
public class IFAS {
void method(boolean isTrue) {
_value = (isTrue ? 0 : 1); // compact expression.
}
private int _value = 0;
编辑推荐:
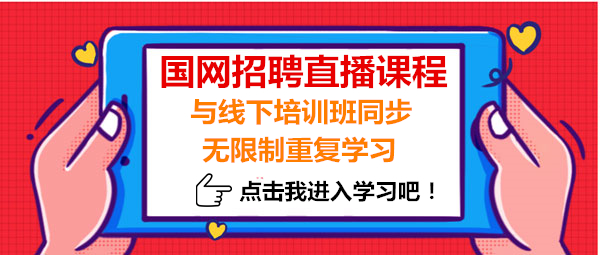
温馨提示:因考试政策、内容不断变化与调整,长理培训网站提供的以上信息仅供参考,如有异议,请考生以权威部门公布的内容为准! (责任编辑:长理培训)
点击加载更多评论>>